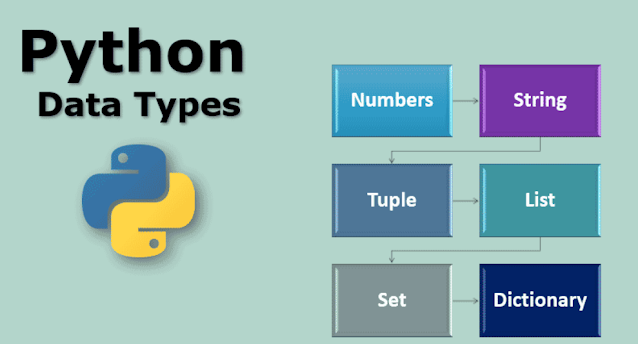
Python- Data Types
Data Types in Python:
The
following are data types in the python programming language...
· Numbers, Strings, Boolean, Print Formatting, Lists, Tuples, Dictionaries, Sets, Comparison Operators, If, elif and else statements, For & While loops, Range, List Comprehension, Lambda, Map and Filter, Functions.
Now let us
see one by one...
------------------------------------------------------------------------
Numbers: All integers and can be defined as int.
Eg: a=3
Type(a)
Output: int
------------------------------------------------------------------------
Strings: Sentences and can be defined as str.
Eg: he =
“Jashwanth is good boy”
Type(he)
Output: str
------------------------------------------------------------------------
Boolean: It only returns True or False. Also, 0 or 1.
Here 1 means true where as 0 means false. It can be defined as bool
Eg: ab=True
Type(ab)
Output: bool
------------------------------------------------------------------------
Print Formatting: we will be using .format method for
printing values. The below example will make this easy to understand.
Eg:
print(“Jashwanth is {} and he loves {}” .format(“Good Boy”, “Gaming”))
Output: Jashwanth is Good Boy and he loves
Gaming
You can
even take variables and use them in format to print.
------------------------------------------------------------------------
Lists: Lists are mutable (Changable). We
can add or delete items by using index. List is enclosed with items and [].
Eg:
L=[“hi”, “bye”]
Type(L)
Output: List
Few More Examples:
L.append("jas")
print(L)
[“hi”,”bye”,”jas”]
L[0]
“hi”
------------------------------------------------------------------------
Tuples: Tupes are immutable (Un Changable).
Enclosed with (). Unable to add or
delete item in tuple.
Eg:
t=(1,2,3)
Type(t)
Output: tuple
NOTE: The
key difference between list and tuple is...
Lists are
mutable and tuples are immutable
Can add or
delete any item out through indexing in lists but not in tuple.
------------------------------------------------------------------------
Dictionaries: It contains keys and values and
arranged in {} brackets.
Eg: dic={"jas":"Reddy",
"Good":"Boy"}
Type(jas)
Output: Dict
dic["jas"]
= Reddy
------------------------------------------------------------------------
Sets: It contains only unique set of elements. If
there is any duplicate item in it, It will consider only once.
Eg: see={"kk","ll","mm","nn","nn"}
Print(see)
Output: ={"kk","ll","mm","nn"}
------------------------------------------------------------------------
Comparison Operators: Helps to compare two or more.
Eg: ==,
<, >, <=, >=, !=
Code:
j=[1,2,3]
k=[1,2,3]
if j==k:
print("TRUE")
else:
print("FALSE")
Output:
TRUE
------------------------------------------------------------------------
If, Elif and Else Statements: Used to perform decision making,
These are known as conditional operators.
Example:
def
fun(age):
if age>18 and age<60:
print("Major")
elif age>60:
print("OLD")
else:
print("Minor")
age=int(input())
fun(age)
Output:
17
Minor
------------------------------------------------------------------------
For & While Loops:
For loop is
used for definite purposes whereas while is used for infinite loops.
FOR Loop Example:
lisss=[1,2,3,4,5]
for item in
lisss:
if item % 2 ==0:
print(item)
Output:
2 4
WHILE Loop
Example:
def
doo(num,word):
while num<5:
print(word)
num+=1
num=int(input())
word=str(input())
doo(num,word)
Output:
1
ll
ll
ll
ll
ll
------------------------------------------------------------------------
Range:
Range is
inbuilt function in python. It print values starting from given starting number
and ending number. For instance range (0,5) means it will print numbers
starting from 0 and ends with 5.
Eg:
for i in
range(0,5):
print(i)
Output: 0,1,2,3,4
------------------------------------------------------------------------
List Comprehension:
Creating
new list by using existing values. Without using list comprehension we have to
write conditional statements inside by using for loop.
Without using List Comprehension:
Example:
loki=[1,2,3,4,5]
for num in
loki:
if num % 2 !=0:
print(num)
Output:
1,3,5
------------------------------------------------------------------------
With using List Comprehension:
Example:
applee=[11,22,33,44,55,66,77,88]
newlis=
[num**2 for num in applee]
print(newlis)
Output:
[121, 484, 1089, 1936, 3025, 4356, 5929, 7744]
------------------------------------------------------------------------
Lambda:
A lambda is
anonymous function. It only takes one argument.
Example:
j=lambda a:
a+10
print
(j(1))
Output: 11
------------------------------------------------------------------------
Map: It is a built in function in python. If we use
map, It will iterates each item.
For
Example:
ja=[11,12,13]
list(map(lambda
num: num*2, ja))
Output:
[22, 24, 26]
------------------------------------------------------------------------
Filter: It filters and take out the items in list as
per condition.
Example:
fruits=["apple","mango","banana","grapes","guva","cherry"]
print(list(filter(lambda
item: item[0]=="g", fruits)))
Output: ['grapes', 'guva']
------------------------------------------------------------------------
Functions:
A function
is a block of code, It only runs when it is called.
Example:
def
mail(a):
doop = a.split("@")
print(doop[1])
a=input("enter
mail:")
mail(a)
Output:
enter mail:admin@boldevs.in
boldevs.in